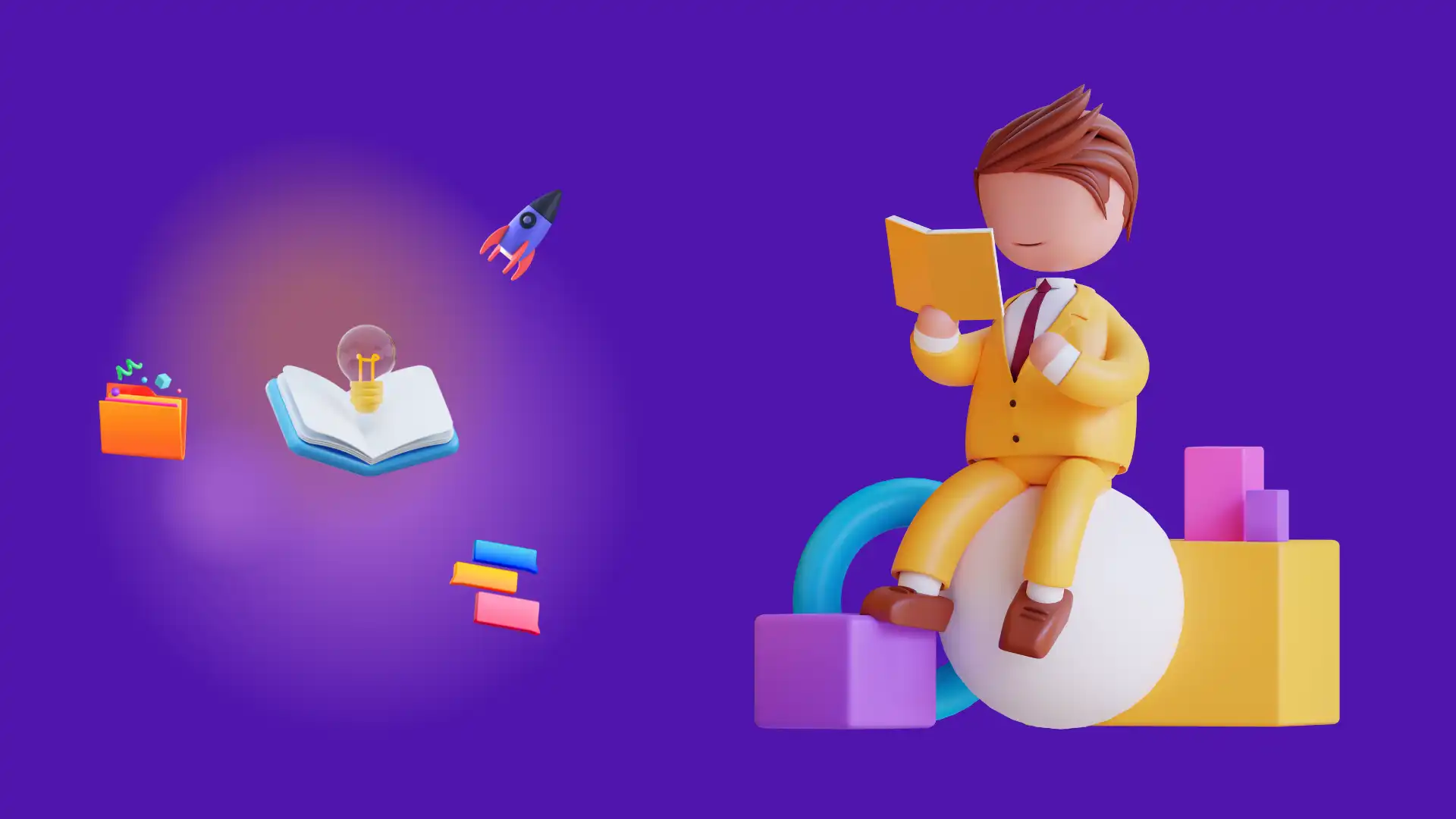
The Complete Guide to Programming Basics for Career Changers
Switching to a programming career from a non-technical background can feel challenging at first. But, it’s definitely doable. Once you learn programming fundamentals, many new career opportunities open up for you.
This guide will walk you through the programming fundamentals. We will cover key concepts and help you to suuceed in your programming career.
Table of Contents
What is Programming?
Programming is a step-by-step process to complete a task by breaking it into smaller tasks. These tasks are called instructions and must follow specific rules.
We use programming languages to write these instructions. Programming helps in communication between humans and computers. It allows you to build web applications, mobile apps, games, and more.
Key Programming Concepts You Must Know
Before you start writing code, it's important to grasp a few key concepts that are used in almost every programming language. These concepts form the foundation of your programming knowledge.
1. Variables and Data Types
A variable is a container for storing data. Think of it like a box where you can keep different kinds of information, such as numbers or words.
In programming, we use data types to define what kind of data a variable can store. Common data types include:
- Integer: Whole numbers, like
5
or100
. - Float: Decimal numbers, like
3.14
or0.001
. - String: Text, such as
"Hello, World!"
. - Boolean: A value that is either
True
orFalse
.
Note: We will also add JavaScript example soon.
Here’s an example in Python:
name = "Alice" # This is a string`
age = 25 # This is an integer`
height = 5.6 # This is a float`
is_student = True # This is a boolean`
Understanding variables and data types is one of the first steps to writing functional code.
2. Operators
Operators are symbols used to perform operations on variables. They allow you to manipulate data. Some common operators are:
- Arithmetic operators:
+
,-
,*
,/
(used for calculations) - Comparison operators:
==
,>
,<
,!=
(used to compare values) - Logical operators:
and
,or
,not
(used for combining conditions)
Example:
a = 10
b = 5
result = a + b # result is 15
Operators are essential in almost every program, whether you're performing calculations or making decisions based on certain conditions.
3. Conditional Statements (If/Else)
One key element of programming is decision-making. Conditional statements allow you to choose different actions based on certain conditions. The if
statement is used to execute code only if a condition is true.
Example:
age = 20
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
In this example, the program checks if the person is 18 or older. If true, it prints "You are an adult"; otherwise, it prints "You are a minor."
4. Loops
Sometimes, you want to repeat certain tasks. Loops let you do this automatically. There are two main types of loops:
- For loop: Repeats a block of code a specific number of times.
- While loop: Repeats a block of code as long as a condition is true.
Example of a for
loop:
for i in range(5):
print(i)
This loop will print numbers from 0 to 4.
5. Functions
A function is a block of reusable code that performs a specific task. Functions help organize your code into smaller, manageable pieces. You can use a function whenever you need it, which keeps your code clean and efficient.
Here’s an example of a simple function in Python:
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
greet("Bob") # Output: Hello, Bob!
The function greet()
takes a parameter (name
) and prints a greeting. You can call the function as many times as you like with different inputs.
6. Lists
A list is a collection of items. You can store multiple values in a list, such as numbers, strings, or even other lists. Lists are ordered, meaning the items have a specific position (or index).
Here’s an example of a list:
fruits = ["apple", "banana", "cherry"]
You can access individual elements in a list using their index, like this:
print(fruits[0]) # Output: apple
Lists allow you to manage and manipulate data in a simple and organized way.
7. Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a way to organize code into objects. An object is an instance of a class, which is like a blueprint for creating objects. Objects can store data (called attributes) and perform actions (called methods).
Here’s an example of a simple class in Python:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def display_info(self):
print(f"Car make: {self.make}, model: {self.model}")
my_car = Car("Toyota", "Corolla")
my_car.display_info() # Output: Car make: Toyota, model: Corolla
In this example, Car
is a class, and my_car
is an object created from that class. OOP helps structure your code, especially for larger projects.
8. Debugging and Error Handling
Programming often involves solving problems, and errors are a natural part of that process. Debugging is the process of finding and fixing errors in your code.
Python provides error messages that can help you figure out what went wrong. You can also use error handling to manage situations where things might go wrong, preventing your program from crashing.
For example, here's how you can handle an error when dividing by zero:
try:
num = int(input("Enter a number: "))
print(10 / num)
except ZeroDivisionError:
print("You cannot divide by zero!")
except ValueError:
print("Please enter a valid number!")
In this case, if the user enters 0
, the program will print "You cannot divide by zero!" instead of crashing.
How to Keep Improving Your Programming Skills
Once you understand these basics, you need to practice. Programming is a skill that improves with time and repetition. Here are some tips for continuing your learning:
1. Practice Coding Regularly
Consistency is key. Try to code every day, even if it’s just for 20–30 minutes. The more you practice, the faster you’ll improve.
2. Solve Small Problems
Start with small coding problems. These help reinforce your understanding and build your confidence. Websites like HackerRank and LeetCode offer coding challenges to practice.
3. Work on Projects
Once you're comfortable with the basics, try building small projects. They can be as simple as a to-do list app or a basic calculator. Projects help you apply what you’ve learned and teach you new skills.
4. Ask for Help
When you're stuck, don’t hesitate to ask for help. Join programming communities like Stack Overflow or Reddit’s r/learnprogramming. People are usually happy to help beginners.
5. Learn from Mistakes
Don’t be afraid to make mistakes. They are part of the learning process. Each error is an opportunity to learn and grow as a programmer.
Conclusion
Learning programming fundamentals as a career changer is challenging, but it’s also incredibly rewarding. Start with the basics: understand variables, control flow, loops, functions, and objects. Keep practicing, and soon enough, you'll be ready to tackle real-world projects. Stay curious, be patient, and keep coding — your new career is just a few lines of code away!