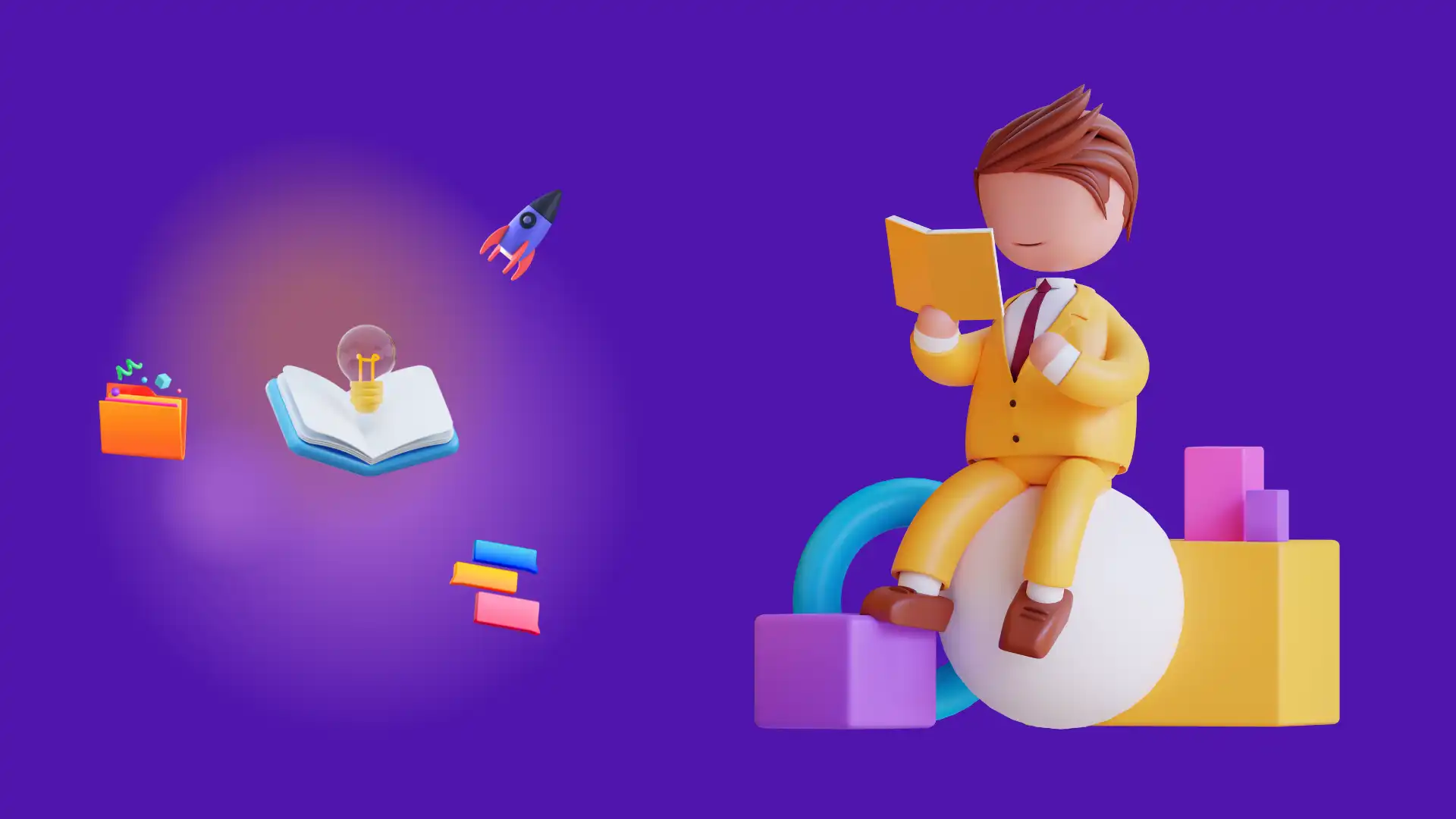
Split String in Python: A Guide to String Splitting Techniques
As a Python programmer, you often come across situations where you need to split a string into smaller parts. Whether it's separating words in a sentence, breaking down a URL, or extracting specific information from a data string, Python provides several methods to split strings effortlessly.
In this article, we'll explore various techniques to split strings in Python and how they can be applied in different scenarios.
Table of Contents
What is a string in Python?
Before we dive into string splitting, let's quickly recap what a string is in Python. In Python, a string is a sequence of characters enclosed within either single quotes ('
) or double quotes ("
). Strings are immutable, meaning they cannot be changed once created. However, you can perform various operations on strings, including splitting them into smaller parts.
Splitting a string using the split()
method
The most straightforward way to split a string in Python is by using the built-in split() method. This method splits a string into a list of substrings based on a delimiter. By default, the delimiter is a space character.
Syntax of the split()
method
- The syntax for using the
split()
method is as follows:
string.split(separator, maxsplit)
- Here, the separator parameter specifies the character or substring at which the string should be split, and the optional maxsplit parameter determines the maximum number of splits to be performed.
Example of splitting a string into a list of substrings
- Let's consider an example to understand how the split() method works:
sentence = "Hello, how are you today?"
words = sentence.split()
print(words)
- output
['Hello,', 'how', 'are', 'you', 'today?']
- In the above example, we split the string sentence into a list of words using the split() method without providing any separator. The resulting list words contains each word as a separate element.
Splitting a string based on a delimiter
Apart from using the default space character as the delimiter, you can specify a custom delimiter to split the string at specific points.
Using a space as a delimiter
- To split a string into words, you can simply use a space character as the delimiter. Consider the following example:
sentence = "Hello, how are you today?"
words = sentence.split(" ")
print(words)
['Hello,', 'how', 'are', 'you', 'today?']
Using a specific character as a delimiter
If you want to split a string at a specific character, you can specify that character as the delimiter. Let's say we have the following URL:
url = "https://www.example.com/products/12345"
To extract the product ID from the URL, we can split the string based on the forward slash (/) delimiter:
product_id = url.split("/")[-1]
print(product_id)
output
12345
In this example, the string url is split into different sections based on the forward slash delimiter, and we extract the last element of the resulting list, which represents the product ID.
Splitting a string into a list of words
To split a string into a list of words, you can use the split() method without specifying any delimiter. Consider the following example:
sentence = "Python programming is fun!"
words = sentence.split()
print(words)
output
['Python', 'programming', 'is', 'fun!']
Here, the string sentence is split into a list of words, with each word as a separate element.
Splitting a string into multiple variables
Python provides a convenient way to split a string into multiple variables using the concept of unpacking.
Using the unpacking feature
To split a string into multiple variables, you need to assign the individual parts of the string to separate variables. This can be achieved using the unpacking feature in Python.
Example of splitting a string into multiple variables
Consider the following example:
name, age, city = "John,25,New York".split(",")
print("Name:", name)
print("Age:", age)
print("City:", city)
output
Name: John
Age: 25
City: New York
In this example, the string "John,25,New York" is split into three parts based on the comma delimiter. The resulting substrings are assigned to the variables name, age, and city, respectively.
Splitting a string using regular expressions
In more complex scenarios, where the splitting requirements are based on a pattern rather than a fixed delimiter, you can utilize regular expressions to split a string.
Overview of regular expressions
Regular expressions, often referred to as regex, are powerful patterns used for pattern matching and string manipulation. They allow you to define complex rules for splitting strings based on specific patterns.
Using the re module to split a string
Python provides the re module, which contains functions for working with regular expressions. The re.split() function allows you to split a string using a regular expression pattern.
Example of splitting a string using regular expressions
Let's say we have the following string:
data = "apple,banana-cherry.orange"
To split the string at commas (,), hyphens (-), and dots (.), we can use the re.split() function:
import re
result = re.split("[,-.]", data)
print(result)
output
['apple', 'banana', 'cherry', 'orange']
Here, we split the string data using the regular expression [,-.], which matches any comma, hyphen, or dot character. The resulting substrings are stored in the result list.
Conclusion
Splitting strings is a fundamental operation in Python, and understanding the various techniques available can greatly enhance your string manipulation capabilities. In this article, we explored different methods to split strings in Python, including the split()
method, custom delimiters, multiple variable splitting, and the use of regular expressions. By incorporating these techniques into your code, you can efficiently extract valuable information from strings and manipulate them to suit your specific needs.
Frequently Asked Questions (FAQs)
Q1: Can I split a string into a list of characters in Python?
- Yes, you can split a string into a list of characters in Python. One way to achieve this is by using list comprehension. Here's an example:
string = "Hello"
characters = [char for char in string]
print(characters)
- output
['H', 'e', 'l', 'l', 'o']
- In this example, the string "Hello" is split into a list of individual characters.
Q2: Is the split() method case-sensitive?
- Yes, the split() method in Python is case-sensitive. It treats uppercase and lowercase characters as distinct. For example:
sentence = "Hello world"
words = sentence.split()
print(words)
- Output:
['Hello', 'world']
- In this case, "Hello" and "hello" would be considered different words if present in the string.
Q3: Can I split a string into a limited number of substrings?
- Yes, the split() method allows you to specify the maximum number of splits using the maxsplit parameter. For example:
sentence = "I like to eat apples and oranges."
words = sentence.split(" ", 3)
print(words)
- Output:
['I', 'like', 'to', 'eat apples and oranges.']
- In this example, the string is split at the first three occurrences of the space character.
Q4: How can I handle leading or trailing whitespace when splitting a string?
- The
split()
method automatically handles leading and trailing whitespace by ignoring them. It splits the string at the spaces between words, discarding any extra whitespace. Here's an example:
string = " Python programming is fun! "
words = string.split()
print(words)
- Output:
['Python', 'programming', 'is', 'fun!']
- In this case, the leading and trailing whitespace is removed, and only the words are included in the resulting list.
Q5: Can I split a string into a list of lines or paragraphs?
- Yes, you can split a string into a list of lines or paragraphs by using the split() method with the newline character (\n) as the delimiter. Here's an example:
text = "This is line 1.\nThis is line 2.\n\nThis is line 4."
lines = text.split("\n")
print(lines)
- Output:
['This is line 1.', 'This is line 2.', '', 'This is line 4.']
- In this example, the string is split at each occurrence of the newline character, resulting in a list of lines.