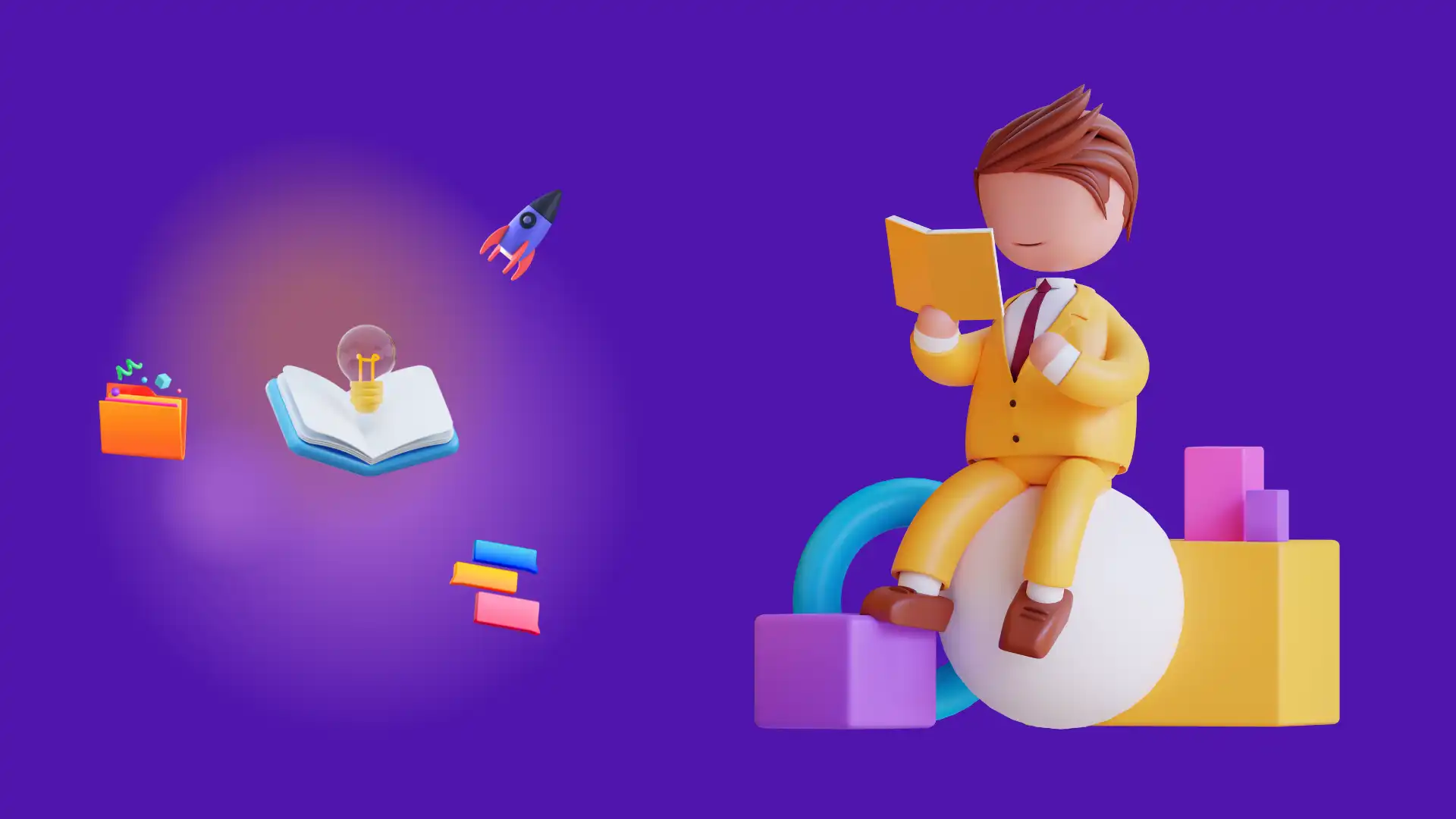
List, Tuple, Set, and Dictionary: Core Data Structures in Python
Python, a popular and versatile programming language, provides a rich set of data structures to efficiently store and manipulate data. List, tuple, set, and dictionary are fundamental data structures that form the backbone of many Python programs. In this blog post, we will delve into each of these data structures, understanding their characteristics, use cases, and the operations that can be performed on them.
Table of Contents
List
The list contains a group of elements. Each element in the list is separated by a comma. We can store elements of different data types like integers, strings, and boolean. Consider the following example:
list_in_python = ["apple", "samsung", "banana", 23, "50%", true]
- In the above code block, we are using square brackets
[]
to store elements in the list namedlist_in_python
. - In the
list_in_python
, we are storing brand name (i.e. apple, samsung) having string data types, a number 23 having integer data types, and a boolean (i.e. true and false). So, a list can store the elements of any data type. That's why the list in Python is heterogeneous. - We can change elements in the list, and add or remove elements from the list. So, the list in Python is mutable.
- Lists store data in a particular order. That means what we will add first, will be stored first
- We can also store duplicate values in the list.
Access elements in the list
my_travelling_preferences = ["Flight","Train","Ship"]
- Consider the above example, We declare a list named
my_travelling_preferences
. We can use the index of elements to access elements in the listmy_travelling_preferences
as shown below:
print(my_travelling_preferences[0]) # output: Flight
print(my_travelling_preferences[1]) # output: Train
print(my_travelling_preferences[2]) # output: Ship
Important List methods
Here is some method of the list that helps to insert, remove and reverse the elements of the list my_travelling_preferences
:
append()
:
my_travelling_preferences = ["Flight","Train","Ship"]
my_travelling_preferences.append("Bike")
print(my_travelling_preferences) # output: ['Flight', 'Train', 'Ship', 'Bike']
insert()
:
my_travelling_preferences = ["Flight", "Train", "Ship"]
my_travelling_preferences.insert(1, "Car")
print(my_travelling_preferences) # output: ['Flight', 'Car', 'Train', 'Ship']
pop()
:
my_travelling_preferences = ["Flight", "Train", "Ship"]
my_travelling_preferences.pop()
print(my_travelling_preferences) # output: ['Flight', 'Train']
sort()
:
my_travelling_preferences = ["Flight", "Train", "Ship"]
my_travelling_preferences.sort()
print(my_travelling_preferences) # output: ['Flight', 'Ship', 'Train']
reverse()
:
my_travelling_preferences = ["Flight", "Train", "Ship", "Car"]
my_travelling_preferences.reverse()
print(my_travelling_preferences) # output: ['Car', 'Ship', 'Train', 'Flight']
Tuple
Just like a list, Tuple contains one or more elements. Each element in the tuple is separated by a comma. We can store elements of various data types like integers, strings, and boolean. Consider the following example:
tuple_in_python = ("apple", "samsung", "banana", 23, "50%", true)
- In the above code block, we are using parentheses
()
to store elements in the list namedtuple_in_python
. - We can not change elements in the tuple. So, tuple in python is immutable.
Note: If there is only one element in the tuple, then we must add a trailing comma. Otherwise, that one element would be a string. For example:
tuple_in_python_without_trailing_comma = ("apple") # the output will be a string: apple print(tuple_in_python_without_trailing_comma) tuple_in_python_with_trailing_comma = ("apple",) # the output will be a tuple: ('apple',) print(tuple_in_python_with_trailing_comma )
Access the Tuple elements
my_integers = (1, 2, 3, 4, 5)
- Consider the above example, We declare a tuple named
my_integers
. We can use the index of elements to access elements in the tuplemy_integers
as shown below:
print(my_integers[0]) # output: 1
print(my_integers[1]) # output: 2
print(my_integers[3]) # output:4
Set
The Set also contains one or more elements. We can store one or more elements of different data types like integers, strings, and boolean. It stores elements in an unordered format. The set doesn't allow duplicate elements.
All the elements in the Set()
must be hashable. Also, a List can not be an element in the set.
random_set = {1, (2, 3), 4, 5, "hello", ("apple", "samsung"), "mango"}
Access the Set elements
random_set = {1, (2, 3), 4, 5, "hello", ("apple", "samsung"), "mango"}
# iterate the set elements
for set in random_set:
print(set)
# using `in` keywords
if "hello" in random_set:
print("Found the string: hello")
else:
print("Not Found the string: hello")
Important Set methods
add()
:
random_set = {1, (2, 3), 4, 5, "hello", ("apple", "samsung"), "mango"}
random_set.add("blue")
print(random_set) # output: {'hello', 1, (2, 3), ('apple', 'samsung'), 4, 5, 'blue', 'mango'}
remove()
: Remove given element from set. Raise KeyError if not present
random_set = {1, (2, 3), 4, 5, "hello", ("apple", "samsung"), "mango", "blue"}
random_set.remove("blue")
print(random_set) # outpur: {1, 4, 5, 'mango', (2, 3), 'hello', ('apple', 'samsung')}
clear()
:
random_set = {1, (2, 3), 4, 5, "hello", ("apple", "samsung"), "mango"}
random_set.clear()
print(random_set) # output: Empty set -> Set()
Dictionary
The dictionary is a collection of elements where each element has key and value pairs. Element keys in the dictionary must be unique. Keys in the dictionary should be hashable.
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
Access the Dictionary elements
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
print(random_dictionary["one"]) # output: 1
print(random_dictionary["brand"]) # output: ('apple', 'samsung')
print(random_dictionary["fruits"]) # output: ['mango', 'apple']
Important Dictionary methods
keys()
:
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
print(random_dictionary.keys()) # output: 1
values()
:
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
print(random_dictionary.values()) # output: 1
items()
:
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
print(random_dictionary.items()) # output: 1
update()
:
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
random_dictionary.update({"one": 1.0})
print(random_dictionary) # output: 1
get()
:
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
print(random_dictionary.get("fruits")) # output: 1
clear()
: — remove all elements from dict
random_dictionary = {"one":1, "two":(2, 3), "string": "hello", "brand":("apple", "samsung"), "fruits":["mango", "apple"]}
random_dictionary.clear()
print(random_dictionary) # output: 1
Conclusion
- The list in Python is dynamic, ordered, mutable, and can store duplicate values.
- Tuple in Python is ordered, and immutable but mutable elements inside tuple can be changed.
- The set is unordered and only has hashable elements. The set does not contain duplicate
- The dictionary contains hashable elements with key and value pairs
- For fast access and manipulation, we should use set and dictionary because elements in set and dictionary have unique identifiers